LeetCode算法笔记–Day34
21. Merge Two Sorted Lists
题目:
Merge two sorted linked lists and return it as a new sorted list. The new list should be made by splicing together the nodes of the first two lists.
example
input: 1->2->4, 1->3->4
output: 1->1->2->3->4->4
My Answer:
方法一:递归
- 时间复杂度:O(n + m)
- 空间复杂度:O(n + m)
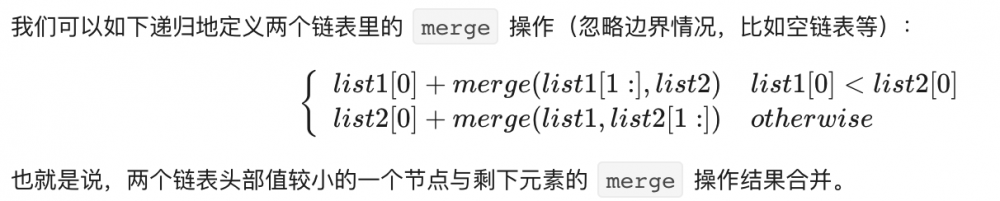
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
|
var mergeTwoLists = function(l1, l2) { if (l1 === null) { return l2; } else if (l2 === null) { return l1; } else if (l1.val < l2.val) { l1.next = mergeTwoLists(l1.next, l2); return l1; } else { l2.next = mergeTwoLists(l1, l2.next); return l2; } };
|
方法二:迭代
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| /** * Definition for singly-linked list. * function ListNode(val, next) { * this.val = (val===undefined ? 0 : val) * this.next = (next===undefined ? null : next) * } */ /** * @param {ListNode} l1 * @param {ListNode} l2 * @return {ListNode} */ var mergeTwoLists = function(l1, l2) { let preHead = new ListNode(-1); let _pre = preHead;
while(l1 != null && l2 != null){ if(l1.val < l2.val){ _pre.next = l1; l1 = l1.next; }else{ _pre.next = l2; l2 = l2.next; } _pre = _pre.next; } _pre.next = l1 == null ? l2 : l1; return preHead.next };
|
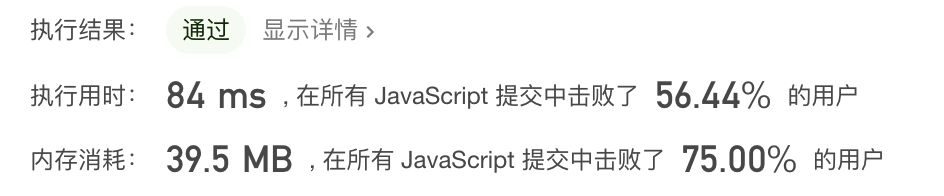